Learning React
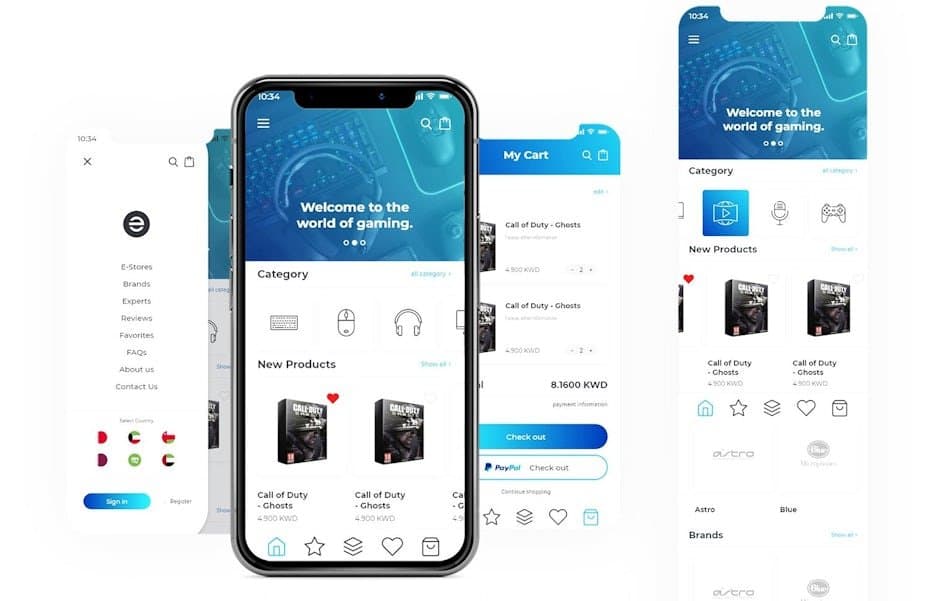

A Beginner’s Guide to React.js: Building Modern Web Applications
🌟 Introduction
In the ever-evolving landscape of web development, React.js has emerged as one of the most powerful and popular libraries for building user interfaces. Created and maintained by Meta (formerly Facebook), React helps developers craft dynamic, responsive, and high-performing web applications with ease.
If you’re curious about what makes React special, how it works, and why developers love it, this blog post is for you!
🚀 What is React.js?
At its core, React.js is an open-source JavaScript library designed for building user interfaces (UIs)—especially for single-page applications (SPAs). Unlike traditional approaches where the whole page reloads when data changes, React updates only the necessary parts of the UI. This makes apps faster and more efficient.
React focuses on the “View” in the MVC (Model-View-Controller) architecture. Its main goal is to help developers create reusable UI components that can handle data changes without reloading the entire page.
🔑 Key Features of React.js
- đź§© Component-Based Architecture
React apps are built using components—small, reusable pieces of code that define parts of the UI. Think of components as LEGO blocks that can be combined to build complex interfaces. - ⚡ Virtual DOM (Document Object Model)
React uses a Virtual DOM to optimize performance. Instead of updating the real DOM directly (which is slow), React updates a lightweight copy of the DOM first, compares it with the previous version, and applies changes only where necessary. - 🔄 One-Way Data Binding
Data flows in one direction, making it easier to debug and maintain applications. The UI is updated automatically when the data changes. - 📦 JSX (JavaScript XML)
React uses JSX, a syntax extension that lets you write HTML-like code within JavaScript. It’s intuitive, readable, and helps structure UI components clearly. - 🌍 Strong Community Support
Being open-source and widely adopted, React has a vibrant community. This means plenty of tutorials, tools, libraries, and third-party packages to speed up development.
🏗️ How Does React Work?
React breaks down UIs into small, manageable components. Here’s a simplified breakdown:
- Components:
Each component is a JavaScript function or class that returns a piece of UI (usually written in JSX). For example:jsxCopyEditfunction Welcome(props) { return <h1>Hello, {props.name}!</h1>; }
- Rendering:
Components are rendered to the DOM using theReactDOM.render()
method. React efficiently updates the DOM using its Virtual DOM algorithm.jsxCopyEditReactDOM.render(<Welcome name="Alice" />, document.getElementById('root'));
- State & Props:
- Props (short for properties) are read-only data passed to components.
- State is mutable data managed within components to handle dynamic changes.
- Lifecycle Methods (for Class Components):
React provides lifecycle methods likecomponentDidMount()
andcomponentDidUpdate()
to handle events at different stages of a component’s life.
📝 Why Choose React?
- Performance: Virtual DOM makes React fast, even for large applications.
- Reusability: Build components once and reuse them across different parts of the app.
- Flexibility: Works well with other libraries and frameworks.
- SEO-Friendly: React can be rendered on the server side, making it better for search engine optimization.
- Strong Ecosystem: Tools like React Router, Redux, and Next.js extend React’s capabilities.
🚀 Getting Started with React
- Setup Your Environment:
Make sure Node.js and npm (Node Package Manager) are installed on your system. - Create a New React App:
Use Create React App, a tool that sets up everything you need. - bashCopyEdit
npx create-react-app my-first-react-app cd my-first-react-app npm start
- Start Building:
Opensrc/App.js
and start editing. The browser will update in real-time as you save changes.
⚡ A Simple React Example
Here’s a basic React component that displays a counter:
jsxCopyEditimport React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<h1>Count: {count}</h1>
<button onClick={() => setCount(count + 1)}>Increase</button>
<button onClick={() => setCount(count - 1)}>Decrease</button>
</div>
);
}
export default Counter;
This small app demonstrates how React handles state and updates the UI dynamically.
🌟 Final Thoughts
React.js isn’t just another JavaScript library—it’s a game-changer for web development. Its flexibility, performance, and strong community support make it the go-to choice for developers building modern web applications.
Whether you’re developing a simple to-do app or a complex enterprise dashboard, React provides the tools and architecture you need to succeed.
🙋 What’s Next?
- Learn React Hooks: Dive deeper into features like
useEffect
,useReducer
, and custom hooks. - Explore React Router: Manage navigation in your React apps seamlessly.
- Try Next.js: Build server-rendered React applications for better performance and SEO.
Happy coding with React! 💻✨